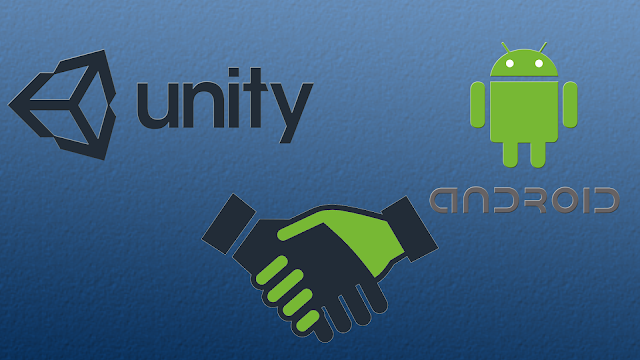
Save Screenshot in Gallery
Make sure you have set Storage Permission to External Storage in Build Settings. To change Storage Permission you need to go File > Build Settings > Player Settings. Now in Inspector window you can change Write Permission under Other Settings section.
You need to just copy and paste below Post to take screenshot and save in Image Gallery. This code must written in subclass of MonoBehaviour.
public void TakeScreenShot() { StartCoroutine(CaptureScreenshotCoroutine(Screen.width, Screen.height)); } private IEnumerator CaptureScreenshotCoroutine(int width, int height) { yield return new WaitForEndOfFrame(); Texture2D tex = new Texture2D(width, height); tex.ReadPixels(new Rect(0, 0, width, height), 0, 0); tex.Apply(); AppStatics._ShowAndroidToastMessage("ScreenShot Saved Successfully"); yield return tex; string path = SaveImageToGallery(tex, "Name", "Description"); } protected const string MEDIA_STORE_IMAGE_MEDIA = "android.provider.MediaStore$Images$Media"; protected static AndroidJavaObject m_Activity; protected static string SaveImageToGallery(Texture2D a_Texture, string a_Title, string a_Description) { using (AndroidJavaClass mediaClass = new AndroidJavaClass(MEDIA_STORE_IMAGE_MEDIA)) { using (AndroidJavaObject contentResolver = Activity.Call("getContentResolver")) { AndroidJavaObject image = Texture2DToAndroidBitmap(a_Texture); return mediaClass.CallStatic("insertImage", contentResolver, image, a_Title, a_Description); } } } protected static AndroidJavaObject Texture2DToAndroidBitmap(Texture2D a_Texture) { byte[] encodedTexture = a_Texture.EncodeToPNG(); using (AndroidJavaClass bitmapFactory = new AndroidJavaClass("android.graphics.BitmapFactory")) { return bitmapFactory.CallStatic("decodeByteArray", encodedTexture, 0, encodedTexture.Length); } } protected static AndroidJavaObject Activity { get { if (m_Activity == null) { AndroidJavaClass unityPlayer = new AndroidJavaClass("com.unity3d.player.UnityPlayer"); m_Activity = unityPlayer.GetStatic("currentActivity"); } return m_Activity; } }
Toast Message code for Android
public static void _ShowAndroidToastMessage(string message) { AndroidJavaClass unityPlayer = new AndroidJavaClass("com.unity3d.player.UnityPlayer"); AndroidJavaObject unityActivity = unityPlayer.GetStatic("currentActivity"); if (unityActivity != null) { AndroidJavaClass toastClass = new AndroidJavaClass("android.widget.Toast"); unityActivity.Call("runOnUiThread", new AndroidJavaRunnable(() => { AndroidJavaObject toastObject = toastClass.CallStatic("makeText", unityActivity, message, 0); toastObject.Call("show"); })); } }