Implement chart with MPAndroidChart
What you need to implement MPAndroidChart in your application is first you need to add dependency into your project.
Add dependancy
project level:
allprojects { repositories { maven { url "https://jitpack.io" } } }
module level:
dependencies { compile 'com.github.PhilJay:MPAndroidChart:v3.0.2' }
Add Line Chart
Now you are ready to use MPAndroidChart in your project. You can implement it with xml or by dynamically adding it in view.
add chart in xml file
< com.github.mikephil.charting.charts.LineChart android:id="@+id/lineChart" android:layout_width="match_parent" android:layout_height="match_parent" />
or add it dynamically
LineChart chart = new LineChart(Context); // get a layout defined in xml RelativeLayout rl = (RelativeLayout) findViewById(R.id.relativeLayout); rl.add(chart); // add the programmatically created chart
Now you have instance of chart, then you can add data in it. To add data you need list of Entry. Entry is model class with x and y points. I have added Entry for demo like below:
private val listData = ArrayList<entry>() listData.add(Entry(0f, 10f)) listData.add(Entry(1f, 20f)) listData.add(Entry(2f, 30f)) listData.add(Entry(3f, 10f)) listData.add(Entry(4f, 40f)) listData.add(Entry(5f, 20f))
Now you need to create LineDataSet object. Modification to chart will comes here. You can specify styling to chart with LineDataSet
val lineDataSet = LineDataSet(listData, getString(R.string.chartLable)) lineDataSet.color = ContextCompat.getColor(this, R.color.colorAccent) lineDataSet.valueTextColor = ContextCompat.getColor(this, android.R.color.white)
At the final step you need to assign LineData to chart. To assign LineData you need to create object of LineData with the help of LineDataSet created in previous step.
val lineData = LineData(lineDataSet) lineChart.data = lineData lineChart.invalidate() //refresh
Bar Chart
to add bar chart you need to use BarEntry. Just modify your list data with this.
private val listData = ArrayList<barentry>() listData.add(BarEntry(1f, 2497f)) listData.add(BarEntry(2f, 12050f)) listData.add(BarEntry(3f, 30000f)) listData.add(BarEntry(4f, 10560f)) listData.add(BarEntry(5f, 14340f)) listData.add(BarEntry(6f, 21234f))
and in xml file add this xml
<com.github.mikephil.charting.charts.BarChart android:id="@+id/chart" android:layout_width="0dp" android:layout_height="0dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" />
Initialise your chart and set data like this
private fun initLineChart() { val xAxis = chart.xAxis xAxis.setDrawLabels(true) xAxis.position = XAxis.XAxisPosition.BOTTOM val xAxisFormatter = DayAxisValueFormatter(chart) xAxis.valueFormatter = xAxisFormatter xAxis.granularity = 1f val rightYAxis = chart.axisRight rightYAxis.setDrawLabels(false) } override fun setChart(listData: ArrayList<barentry>) { val dataSet = BarDataSet(listData, getString(R.string.chartLable)) dataSet.color = ContextCompat.getColor(activity, R.color.colorAccent) dataSet.valueTextColor = ContextCompat.getColor(activity, android.R.color.black) val lineData = BarData(dataSet) chart.setFitBars(true) chart.data = lineData chart.invalidate() //refresh }
This will look like this:
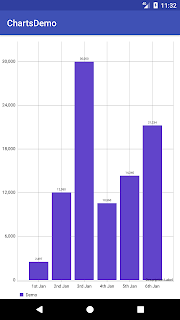
Note that create DayAxiValueFormatter like auther specified. To check it click here
Pie Chart
You can also add PieChart. To add Pie Chart you need to use PieEntry
Init data like this
private val listData = ArrayList<PieEntry>() listData.add(PieEntry(10f, 2497f)) listData.add(PieEntry(20f, 12050f)) listData.add(PieEntry(30f, 30000f)) listData.add(PieEntry(20f, 10560f)) listData.add(PieEntry(10.8f, 14340f)) listData.add(PieEntry(9.2f, 21234f)) getView().setChart(listData)
and set chart as
val dataSet = PieDataSet(listData, getString(R.string.chartLable)) dataSet.color = ContextCompat.getColor(activity, R.color.colorAccent) dataSet.valueTextColor = ContextCompat.getColor(activity, R.color.colorText) dataSet.setColors(intArrayOf(R.color.color1, R.color.color2, R.color.color3), activity) val lineData = PieData(dataSet) chart.data = lineData chart.invalidate() //refresh
In xml you have add PieChart
<com.github.mikephil.charting.charts.PieChart android:id="@+id/chart" android:layout_width="0dp" android:layout_height="0dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" />
Download it from My dropbox link.
If want to know more about it, Visit Github Repository You can also follow author of MPAndroidChart library